前面我们发送和接收的广播全部属于系统全局广播,即发出的广播可以被其他任何应用程序接收到,并且我们也可以接收来自于其他任何应用程序的广播。这样就很容易会引起安全性的问题,比如说我们发送的一些携带关键性数据的广播有可能被其他的应用程序截获或者其他的程序不停地向我们的广播接收器里发送各种垃圾广播。
为了能够简单地解决广播的安全性问题,Android 引入了一套本地广播机制,使用这个机制发出的广播只能够在应用程序的内部进行传递,并且广播接收器也只能接收来自本应用程序发出的广播,这样所有的安全性问题就都不存在了。另外,发送本地广播比起发送系统全局广播效率更高。
本地广播的用法并不复杂,主要就是使用了一个LocalBroadcastManager 来对广播进行管理,并提供了发送广播和注册广播接收器的方法。下面我们就通过具体的实例来演示它的用法。
新建一个CustomReceive类继承自BroadcastReceiver,并重写onReceive()方法,代码如下:
package com.rfstar.localbroadcasttest;
import android.content.BroadcastReceiver;import android.content.Context;import android.content.Intent;import android.os.Bundle;import android.widget.Toast;public class CustomReceiver extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { //获取传递的参数 Bundle bundle=intent.getBundleExtra("data"); String name=bundle.getString("name"); String field=bundle.getString("field"); Toast.makeText(context,"在broadcast应用中接收到广播:"+"接收的广播数据为,名称:"+name+",领域:"+field, Toast.LENGTH_LONG).show(); }}复制代码MainActivity代码如下:
package com.rfstar.localbroadcasttest;
import androidx.appcompat.app.AppCompatActivity;import androidx.localbroadcastmanager.content.LocalBroadcastManager;import android.content.Intent;import android.content.IntentFilter;import android.os.Bundle;import android.view.View;import android.widget.Button;public class MainActivity extends AppCompatActivity {private LocalBroadcastManager localBroadcastManager;private CustomReceiver customReceiver; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);localBroadcastManager=LocalBroadcastManager.getInstance(this); Button send=(Button)findViewById(R.id.send); send.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { //建立一个意图,action为com.rfstar.action.NORMAL_BROADCAST Intent intent=new Intent("com.rfstar.action.NORMAL_BROADCAST"); Bundle bundle=new Bundle(); bundle.putString("name","大鸟科创空间"); bundle.putString("field","科技"); //向意图中加入数据 intent.putExtra("data",bundle); //发送广播,普通广播 localBroadcastManager.sendBroadcast(intent); } }); IntentFilter intentFilter=new IntentFilter(); intentFilter.addAction("com.rfstar.action.NORMAL_BROADCAST"); customReceiver=new CustomReceiver(); localBroadcastManager.registerReceiver(customReceiver,intentFilter); } @Override protected void onDestroy() { super.onDestroy(); localBroadcastManager.unregisterReceiver(customReceiver); }}复制代码
MainActivity对应的布局文件代码如下,创建一个发送广播的Button按钮。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"><Button android:id="@+id/send" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="发送广播" /></LinearLayout>复制代码
这部分代码和我们前面所学的动态注册广播接收者以及发送广播的代码是一样的。只不过现在首先是通过 LocalBroadcastManager 的静态方法 getInstance()得到了LocalBroadcast-Manager 的一个实例,然后用LocalBroadcastManager 对象调用registerReceiver()方法注册广播接收者,再用 LocalBroadcastManager 对象调用 sendBroadcast()方法发送广播。
本地广播是无法通过静态注册的方式来接收的。其实这也完全可以理解,因为静态注册主要就是为了让程序在未启动的情况下也能收到广播,而发送本地广播时,我们的程序肯定是已经启动了,完全不需要使用静态注册的功能,所以也应将 AndroidManifest.xml文件中的静态注册接收者部分删除。
重新运行程序并点击按钮,效果如下图所示。此时其他的应用程序是接收不了这条广播的。
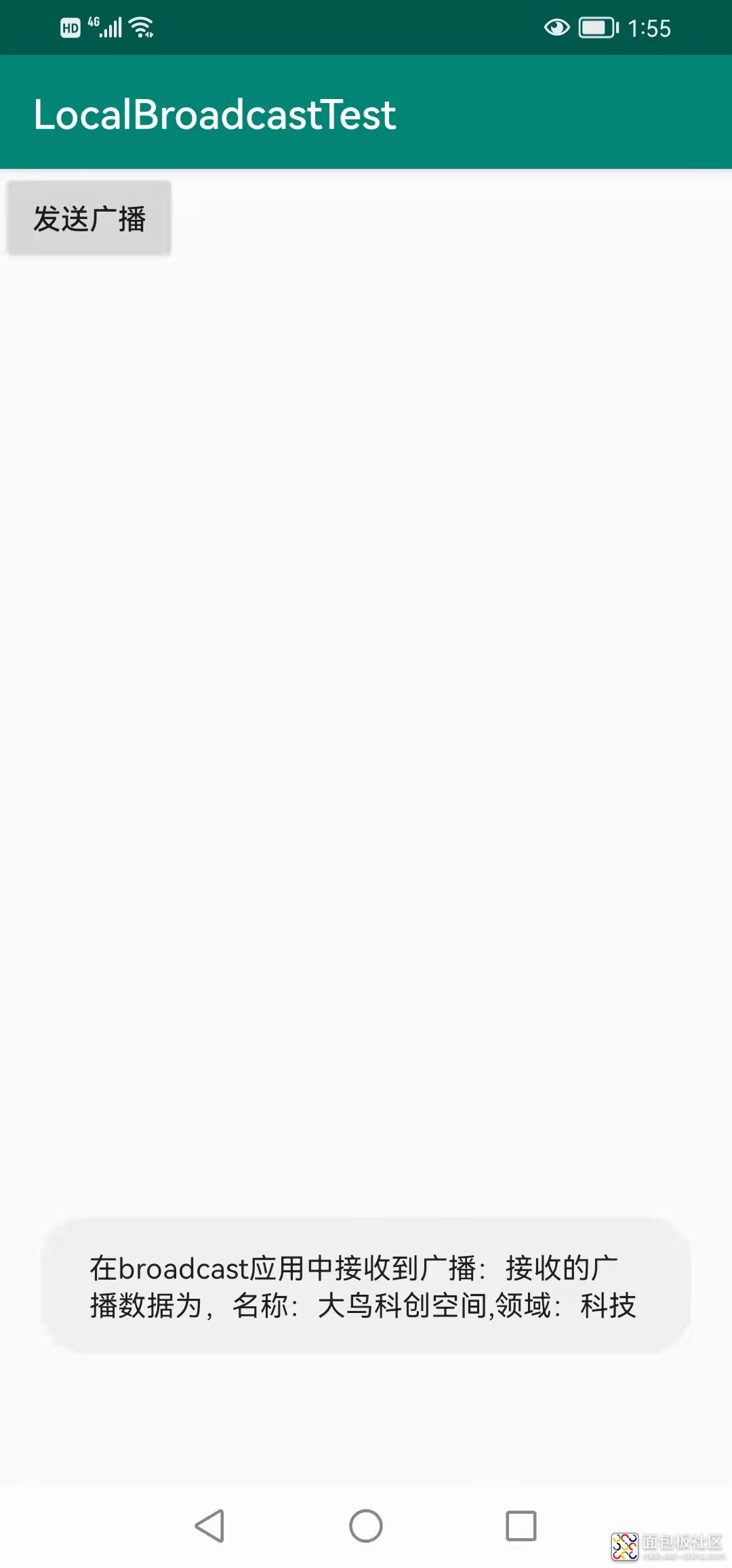
android studio工具及手机模拟器以及更多工程源代码下载请前往微信公众号:大鸟科创空间,回复:android studio即可获取。
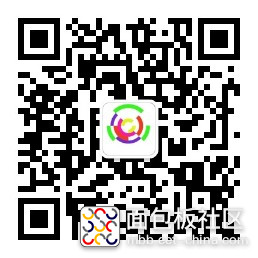
文章评论(0条评论)
登录后参与讨论