voidI2C0_init(void)
{
/* enable I2C0 clock */
rcu_periph_clock_enable(RCU_I2C0);
i2c_software_reset_config(I2C0, I2C_SRESET_SET);
i2c_software_reset_config(I2C0, I2C_SRESET_RESET);
/* connect PB6 to I2C0_SCL */
/* connect PB7 to I2C0_SDA */
gpio_init(GPIOB, GPIO_MODE_AF_OD, GPIO_OSPEED_50MHZ, GPIO_PIN_6|GPIO_PIN_7);
gpio_bit_set(GPIOB, GPIO_PIN_6|GPIO_PIN_7);
gpio_pin_remap_config(GPIO_I2C0_REMAP, DISABLE);
/* I2C clock configure */
i2c_clock_config(I2C0, I2C0_SPEED, I2C_DTCY_2);
/* I2C address configure */
i2c_mode_addr_config(I2C0, I2C_I2CMODE_ENABLE, I2C_ADDFORMAT_7BITS, I2C0_S_ADDR_85RC16);
/* enable I2C0 */
nvic_irq_enable(I2C0_EV_IRQn, 0, 1);
i2c_enable(I2C0);
/* enable acknowledge */
i2c_ack_config(I2C0, I2C_ACK_ENABLE);
i2c_stop_on_bus(I2C0);
}
voideeprom_buffer_read(uint8_t*p_buffer, uint16_tread_address, uint16_tnumber_of_byte)
{
uint8_tAddrValue=0;
AddrValue= (I2C0_S_ADDR_85RC16&0xF0); //
AddrValue|= ((read_address>>7) &0xE); //
/* wait until I2C bus is idle */
while (i2c_flag_get(I2C0, I2C_FLAG_I2CBSY))
;
if (2==number_of_byte)
{
i2c_ackpos_config(I2C0, I2C_ACKPOS_NEXT);
}
/* send a start condition to I2C bus */
i2c_start_on_bus(I2C0);
/* wait until SBSEND bit is set */
while (!i2c_flag_get(I2C0, I2C_FLAG_SBSEND))
;
/* send slave address to I2C bus */
i2c_master_addressing(I2C0, AddrValue, I2C_TRANSMITTER);
/* wait until ADDSEND bit is set */
while (!i2c_flag_get(I2C0, I2C_FLAG_ADDSEND))
;
/* clear the ADDSEND bit */
i2c_flag_clear(I2C0, I2C_FLAG_ADDSEND);
/* wait until the transmit data buffer is empty */
while (SET!=i2c_flag_get(I2C0, I2C_FLAG_TBE))
;
/* enable I2C0*/
i2c_enable(I2C0);
/* send the EEPROM's internal address to write to */
i2c_data_transmit(I2C0, (read_address&0xFF));
/* wait until BTC bit is set */
while (!i2c_flag_get(I2C0, I2C_FLAG_BTC))
;
/* send a start condition to I2C bus */
i2c_start_on_bus(I2C0);
/* wait until SBSEND bit is set */
while (!i2c_flag_get(I2C0, I2C_FLAG_SBSEND))
;
/* send slave address to I2C bus */
i2c_master_addressing(I2C0, AddrValue, I2C_RECEIVER);
if (number_of_byte<3)
{
/* disable acknowledge */
i2c_ack_config(I2C0, I2C_ACK_DISABLE);
}
/* wait until ADDSEND bit is set */
while (!i2c_flag_get(I2C0, I2C_FLAG_ADDSEND))
;
/* clear the ADDSEND bit */
i2c_flag_clear(I2C0, I2C_FLAG_ADDSEND);
if (1==number_of_byte)
{
/* send a stop condition to I2C bus */
i2c_stop_on_bus(I2C0);
}
/* while there is data to be read */
while (number_of_byte)
{
if (3==number_of_byte)
{
/* wait until BTC bit is set */
while (!i2c_flag_get(I2C0, I2C_FLAG_BTC))
;
/* disable acknowledge */
i2c_ack_config(I2C0, I2C_ACK_DISABLE);
}
if (2==number_of_byte)
{
/* wait until BTC bit is set */
while (!i2c_flag_get(I2C0, I2C_FLAG_BTC))
;
/* send a stop condition to I2C bus */
i2c_stop_on_bus(I2C0);
}
/* wait until the RBNE bit is set and clear it */
if (i2c_flag_get(I2C0, I2C_FLAG_RBNE))
{
/* read a byte from the EEPROM */
*p_buffer=i2c_data_receive(I2C0);
/* point to the next location where the byte read will be saved */
p_buffer++;
/* decrement the read bytes counter */
number_of_byte--;
}
}
/* wait until the stop condition is finished */
while (I2C_CTL0(I2C0) &0x0200)
;
/* enable acknowledge */
i2c_ack_config(I2C0, I2C_ACK_ENABLE);
i2c_ackpos_config(I2C0, I2C_ACKPOS_CURRENT);
}
通过文档翻阅写入数据逻辑
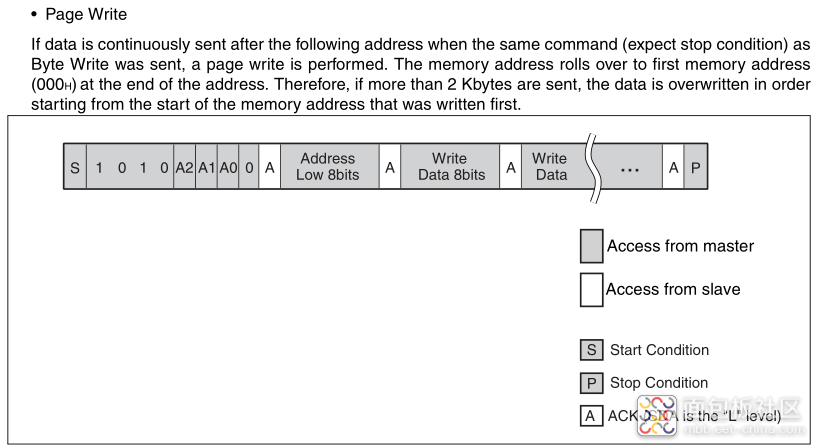
代码实现
voideeprom_buffer_write(uint8_t*p_buffer, uint16_twrite_address, uint16_tnumber_of_byte)
{
uint8_tAddrValue=0;
AddrValue= (I2C0_S_ADDR_85RC16&0xF0); //
AddrValue|= ((write_address>>7) &0xE); //
Eeprom_WREnable();
/* wait until I2C bus is idle */
while (i2c_flag_get(I2C0, I2C_FLAG_I2CBSY))
;
/* send a start condition to I2C bus */
i2c_start_on_bus(I2C0);
/* wait until SBSEND bit is set */
while (!i2c_flag_get(I2C0, I2C_FLAG_SBSEND))
;
/* send slave address to I2C bus */
i2c_master_addressing(I2C0, AddrValue, I2C_TRANSMITTER);
/* wait until ADDSEND bit is set */
while (!i2c_flag_get(I2C0, I2C_FLAG_ADDSEND))
;
/* clear the ADDSEND bit */
i2c_flag_clear(I2C0, I2C_FLAG_ADDSEND);
/* wait until the transmit data buffer is empty */
while (SET!=i2c_flag_get(I2C0, I2C_FLAG_TBE))
;
/* send the EEPROM's internal address to write to : only one byte address */
i2c_data_transmit(I2C0, (write_address&0xFF));
/* wait until BTC bit is set */
while (!i2c_flag_get(I2C0, I2C_FLAG_BTC))
;
/* while there is data to be written */
while (number_of_byte--)
{
i2c_data_transmit(I2C0, *p_buffer);
/* point to the next byte to be written */
p_buffer++;
/* wait until BTC bit is set */
while (!i2c_flag_get(I2C0, I2C_FLAG_BTC))
;
}
/* send a stop condition to I2C bus */
i2c_stop_on_bus(I2C0);
/* wait until the stop condition is finished */
while (I2C_CTL0(I2C0) &0x0200)
;
Eeprom_WRDisable();
}
总结:本次项目中使用的GD32F303读写操作实现,对比之前用的eeprom,速度提高到1M,价格稍微贵了,读写速度提升了
开发工匠 2025-4-22 11:04
eeNick 2025-4-21 11:00