东芝TT_M3HQ开发板支持KEIL、IAR等离线版的IDE开发环境,当然它也有在线编程开发环境,这就是我们所提到的Mbed在线编程工具。
先来看看使用KEIL工具编译官方的工程源码。先要下载安装pack包“Keil.TXZ3_DFP.1.2.0.pack“,在前面的帖子中,串口调试打印工程体验篇一中也有详细介绍,此次主要来看看在”LED_GPIO“工程中的库函数调用方法。
//LED初始化函数void led_initialize(led_t *p_instance) { /*------------------------------*/ /* Timer Processing Disable */ /*------------------------------*/ p_instance->info.timer = TXZ_DISABLE; /*------------------------------*/ /* Port Setting */ /*------------------------------*/ /*--- DATA setting ---*/ { gpio_pinstate_t value; if (p_instance->init.state == LED_STATE_OFF) { value = GPIO_PIN_RESET; } else { value = GPIO_PIN_SET; } if (gpio_write_bit( p_instance->init.p_gpio, p_instance->init.port.group, p_instance->init.port.num, GPIO_Mode_DATA, value ) != TXZ_SUCCESS) { led_error(); } }
复制代码//写参数void led_finalize(led_t *p_instance) { /*------------------------------*/ /* Timer Processing Disable */ /*------------------------------*/ p_instance->info.timer = TXZ_DISABLE; /*------------------------------*/ /* Turn off */ /*------------------------------*/ if (gpio_write_bit( p_instance->init.p_gpio, p_instance->init.port.group, p_instance->init.port.num, GPIO_Mode_DATA, GPIO_PIN_RESET ) != TXZ_SUCCESS) { led_error(); } }
复制代码//亮灭灯执行任务处理函数void led_task(led_t *p_instance) { if (p_instance->init.blink.func == TXZ_ENABLE) { LEDState crt; LEDState nxt; gpio_pinstate_t val = GPIO_PIN_SET; /*---------------*/ /*检查灯的状态 */ /*---------------*/ /*--- Current LED ---*/ if(gpio_read_bit( p_instance->init.p_gpio, p_instance->init.port.group, p_instance->init.port.num, GPIO_Mode_DATA, &val) == TXZ_SUCCESS) { if(val == GPIO_PIN_RESET) { crt = LED_STATE_OFF; } else { crt = LED_STATE_ON; } } /*--- Next LED ---*/ nxt = p_instance->info.state; /*------------------------------*/ /* Turn on/off */ /*------------------------------*/ if (crt != nxt) { gpio_pinstate_t value; if (nxt == LED_STATE_OFF) { value = GPIO_PIN_RESET; } else { value = GPIO_PIN_SET; } if (gpio_write_bit( p_instance->init.p_gpio, p_instance->init.port.group, p_instance->init.port.num, GPIO_Mode_DATA, value ) != TXZ_SUCCESS) { led_error(); } } } }
复制代码//开灯函数void led_turn_on(led_t *p_instance) { /*------------------------------*/ /* Timer Processing Disable */ /*------------------------------*/ p_instance->info.timer = TXZ_DISABLE; /*------------------------------*/ /* Turn on */ /*------------------------------*/ if (gpio_write_bit( p_instance->init.p_gpio, p_instance->init.port.group, p_instance->init.port.num, GPIO_Mode_DATA, GPIO_PIN_SET ) != TXZ_SUCCESS) { led_error(); } /*------------------------------*/ /* Update Information */ /*------------------------------*/ p_instance->info.state = LED_STATE_ON; p_instance->info.counter = 0; /*------------------------------*/ /* Timer Processing Enable */ /*------------------------------*/ if (p_instance->init.blink.func == TXZ_ENABLE) { p_instance->info.timer = TXZ_ENABLE; } } //关灯函数 void led_turn_off(led_t *p_instance) { /*------------------------------*/ /* Timer Processing Disable */ /*------------------------------*/ p_instance->info.timer = TXZ_DISABLE; /*------------------------------*/ /* Turn off */ /*------------------------------*/ if (gpio_write_bit( p_instance->init.p_gpio, p_instance->init.port.group, p_instance->init.port.num, GPIO_Mode_DATA, GPIO_PIN_RESET ) != TXZ_SUCCESS) { led_error(); } /*------------------------------*/ /* Update Information */ /*------------------------------*/ p_instance->info.state = LED_STATE_OFF; p_instance->info.counter = 0; /*------------------------------*/ /* Timer Processing Enable */ /*------------------------------*/ if (p_instance->init.blink.func == TXZ_ENABLE) { p_instance->info.timer = TXZ_ENABLE; } }
复制代码//间隔1ms检测产生的中断句柄void led_1ms_timer_handler(led_t *p_instance) { if (p_instance->info.timer == TXZ_ENABLE) { /*------------------------------*/ /* Counter Update */ /*------------------------------*/ p_instance->info.counter++; /*------------------------------*/ /* LED Update Check */ /*------------------------------*/ if (p_instance->init.blink.func == TXZ_ENABLE) { if (p_instance->info.state == LED_STATE_ON) { if (p_instance->init.blink.interval.on <= p_instance->info.counter) { p_instance->info.state = LED_STATE_OFF; p_instance->info.counter = 0; } } else { if (p_instance->init.blink.interval.off <= p_instance->info.counter) { p_instance->info.state = LED_STATE_ON; p_instance->info.counter = 0; } } } } }
复制代码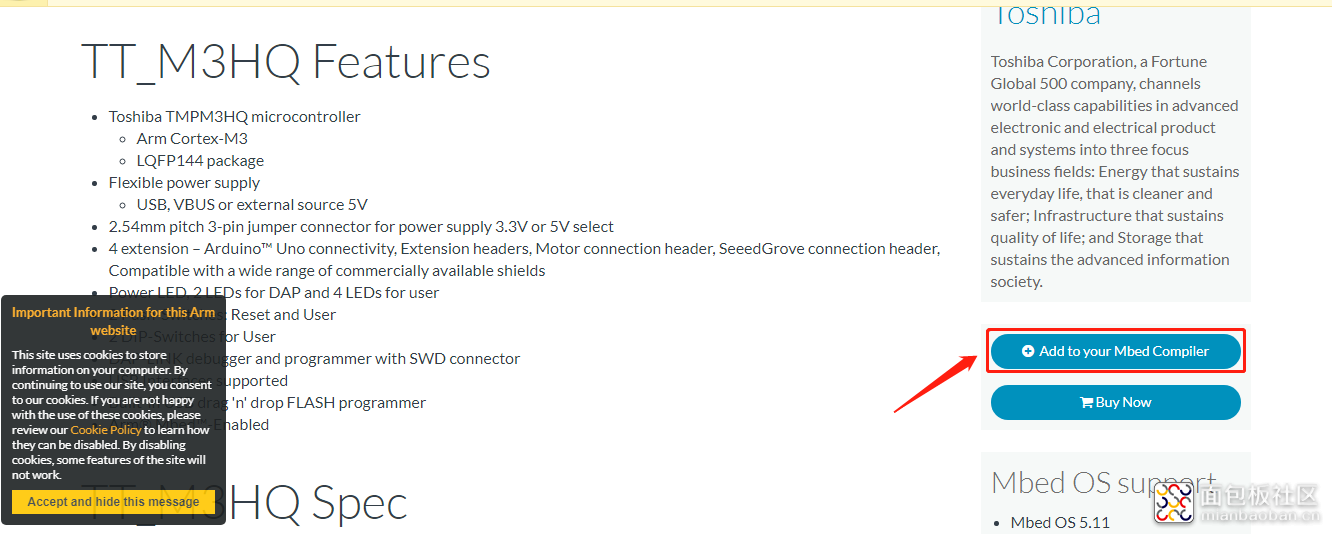
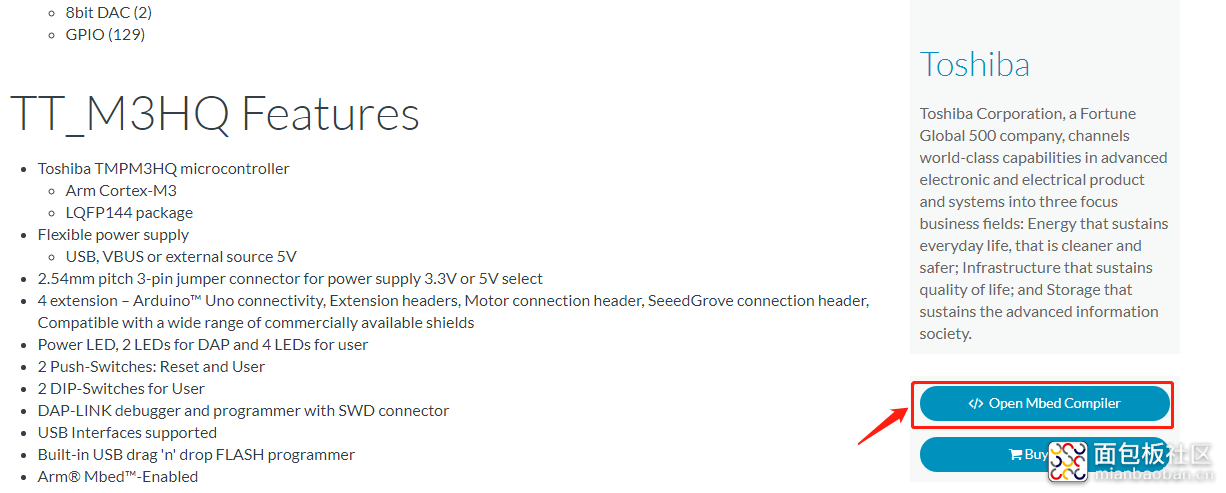
进去后将弹出的对话框关闭
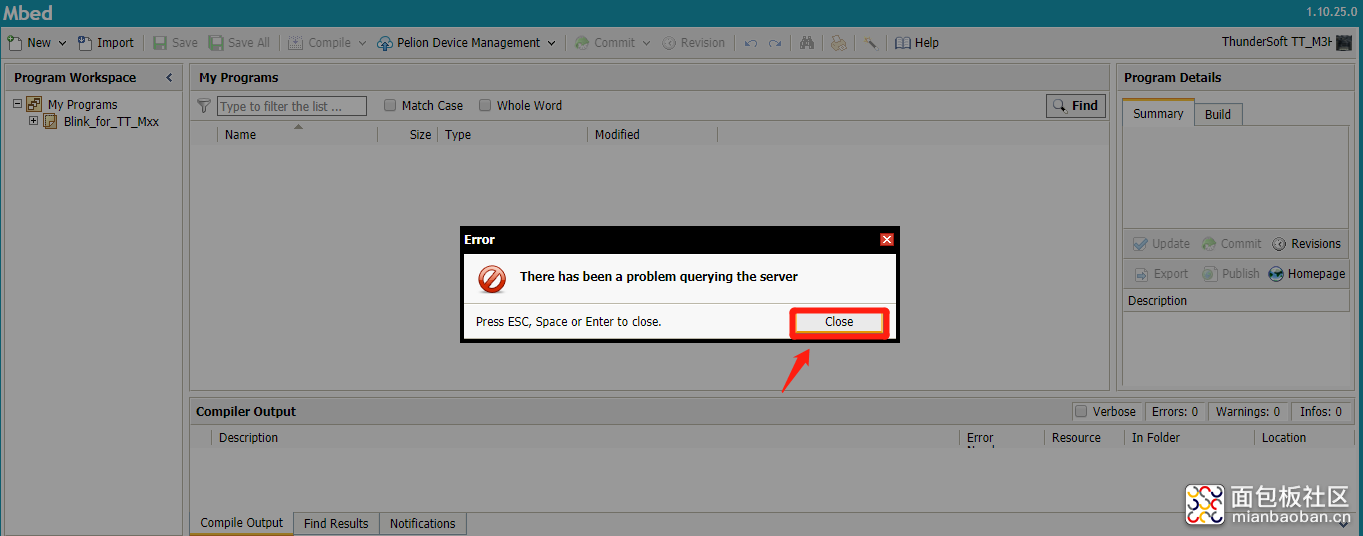
同样的将弹出的对话框关掉(cancle),点击”Import“菜单栏,将官方提供的demo链接加进去,网址:https://os.mbed.com/teams/Thundersoft/code/Blink_for_TT_Mxx/ 然后点击编译,会出现如下图所示:
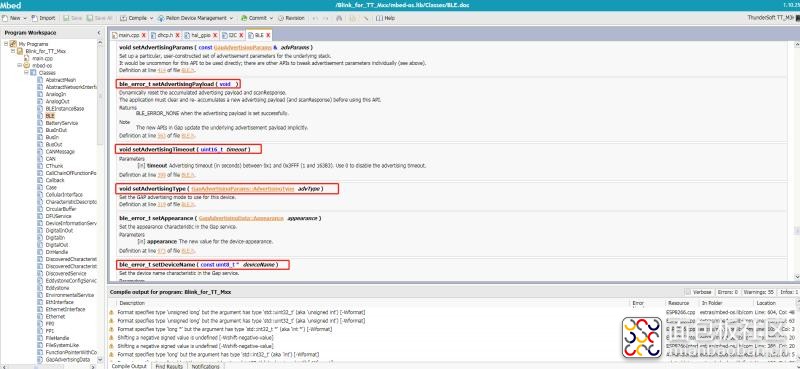
编译成功后截图如下:
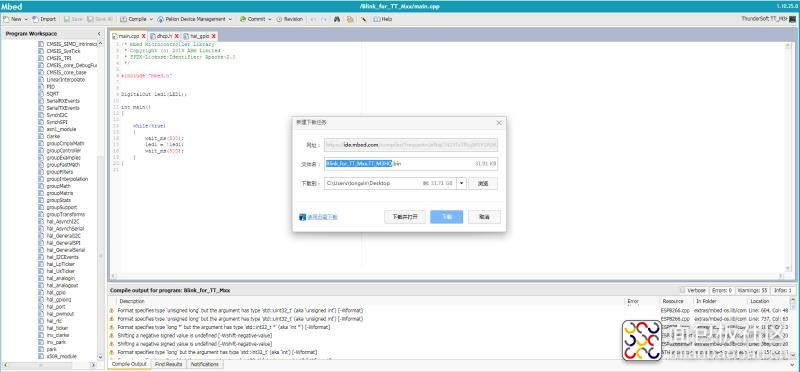
然后将编译好的二进制文件”Blink_for_TT_Mxx.TT_M3HQ.bin“复制到“我的电脑”的移动设备中,如下显示TT_M3HQ的存储空间

拷贝完成后,重新上电,就能看到灯的亮灭状态如我们在程序中设定的一样,有规律的闪烁。此次熟悉了对Mbed的在线编程工具,深刻体会了Mbed的高效便捷式,它不需要我们去安装,搭建编译所需的板级支持包,编译所需的动态库,而且开发人员可以通过网络保存多个版本的工程,这样对工程代码的维护有很好的管理作用。如果Mbed像NXP的在线图形编译工具一样,能够提供很好的图形化编程环境,这样会被更多的开发人员认可,您觉得呢?此次分享就到这里啦,感谢面包社区提供此次评测的机会,后续再对TT_M3HQ展开学习研究,谢谢各位,祝大家中秋节玩得开心,工作顺顺利利,欢度国庆啦。