制作出来的实物图如下:
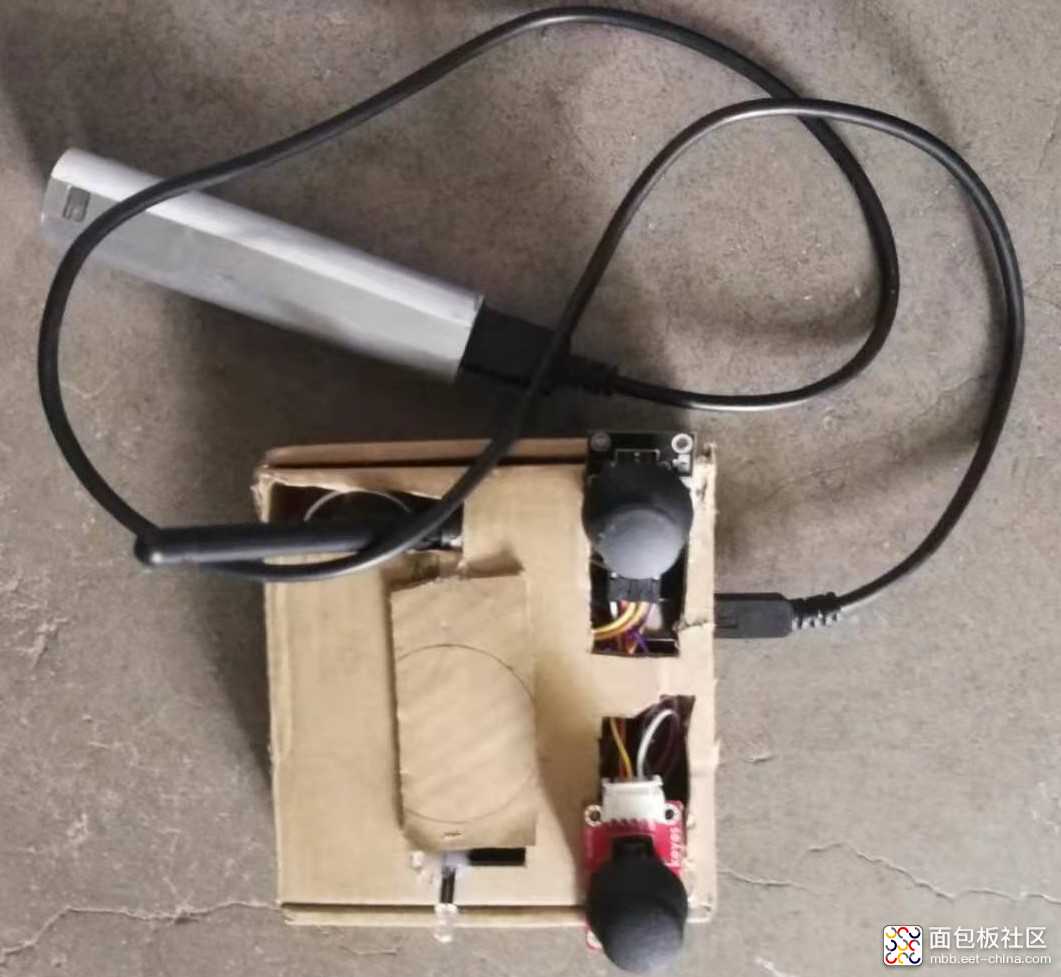
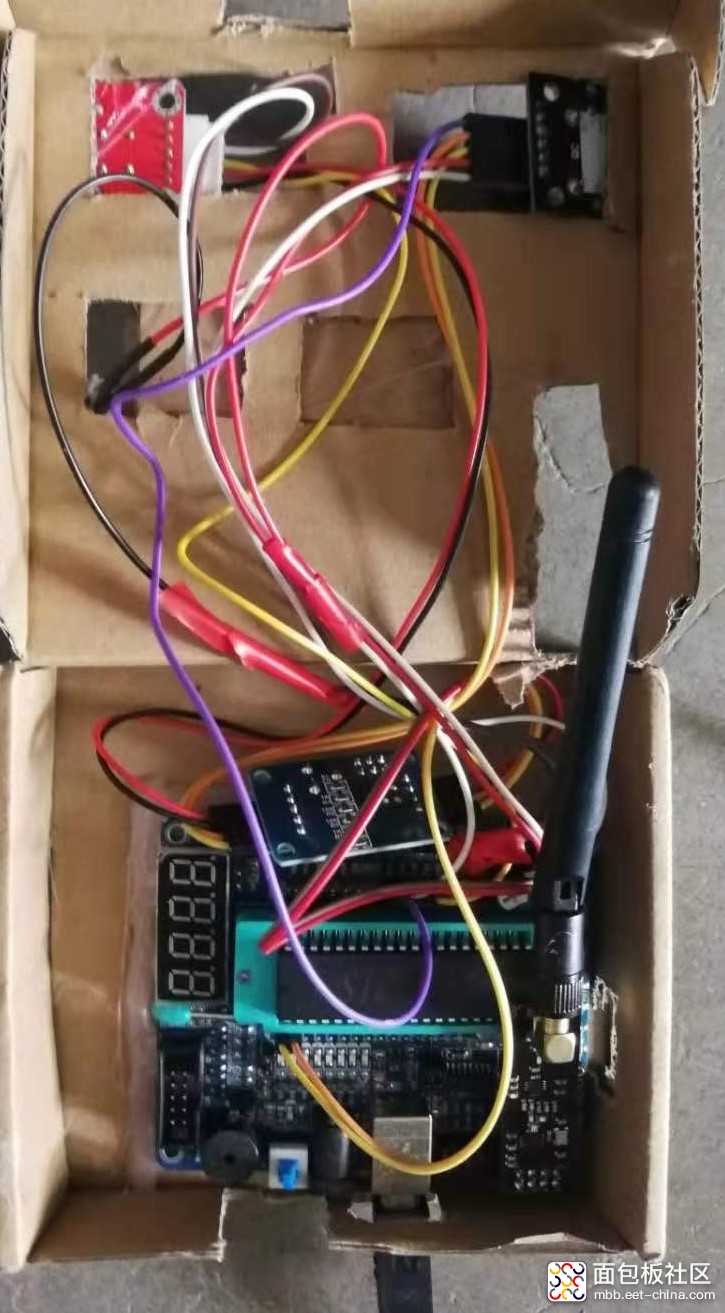
下面介绍一下现在所需的材料,首先该款51单片机并不搭载AD转换芯片,所以得需要另配模块,我所用的即是PCF8591模块,如下图;
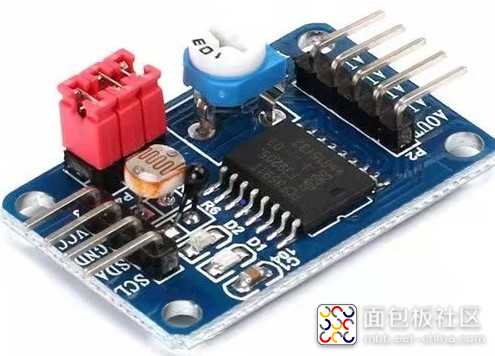
推荐买上图这种的,因为我用过其他种类的pcf8591,多多少少都是程序上不支持,而且该模块可以检测4路AD信号,对于需要做两路控制的遥控器就可以满足条件,话不多说,直接上pcf8591用LCD1602显示程序,我的程序只适用于KR51单片机,需要改程序的根据自己实际需求修改即可;
单片机源程序如下:
#include <reg52.h>#include <intrins.h> #define AddWr 0x90 #define AddRd 0x91 unsigned char AD_CHANNEL=0; unsigned char D[32]; sbit scl=P1^0; sbit sda=P1^1; sbit rs=P2^0; sbit rw=P2^1; sbit lcden=P2^2; bit ack; unsigned char date; void Delay(unsigned char i); void init(); void write_shige(unsigned char b,unsigned char m); ///////////////////////////////////////////////////////////////// void start() { sda=1; _nop_(); scl=1; _nop_();_nop_();_nop_();_nop_();_nop_(); sda=0; _nop_();_nop_();_nop_();_nop_();_nop_(); scl=0; _nop_();_nop_(); } //////////////////////////////////////////////////////////////// void stop() { sda=0; _nop_();_nop_(); scl=1; _nop_();_nop_();_nop_();_nop_();_nop_(); sda=1; _nop_();_nop_();_nop_();_nop_();_nop_(); } //////////////////////////////////////////////////////////////// void SendByte(unsigned char c) { unsigned char i; for(i=0;i<8;i++) { if((c<<i)&0x80) sda=1; else sda=0; _nop_(); scl=1; _nop_();_nop_();_nop_();_nop_();_nop_(); scl=0; } _nop_();_nop_(); sda=1; _nop_();_nop_(); scl=1; _nop_();_nop_();_nop_();_nop_();_nop_(); if(sda==1) ack=0; else ack=1; scl=0; _nop_();_nop_(); } //////////////////////////////////////////////////////////////// unsigned char RevByte() { unsigned char retc=0,i; sda=1; for(i=0;i<8;i++) { _nop_(); scl=0; _nop_();_nop_();_nop_();_nop_();_nop_(); scl=1; _nop_();_nop_(); retc=retc<<1; if(sda==1) { retc=retc+1; } _nop_();_nop_(); } scl=0; _nop_();_nop_(); return(retc); } /////////////////////////////////////////////////////////////// void Ack1(bit a) { if(a==0) { sda=0; } else sda=1; _nop_();_nop_();_nop_(); scl=1; _nop_();_nop_();_nop_();_nop_();_nop_(); scl=0; _nop_();_nop_(); } ////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////// bit PCF8591_DACONVERSION(unsigned char addr,unsigned char channel,unsigned char Val) { start(); SendByte(addr); if(ack==0) return(0); SendByte(0x40|channel); if(ack==0) return(0); SendByte(Val); if(ack==0) return(0); stop(); return(1); } ////////////////////////////////////////////////////////////// bit PCF8591_SendByte(unsigned char addr,unsigned char channel) { start(); SendByte(addr); if(ack==0) return(0); SendByte(0x40|channel); if(ack==0) return(0); stop(); return(1); } //////////////////////////////////////////////////////////// unsigned char PCF8591_RcvByte(unsigned char addr) { unsigned char dat; start(); SendByte(addr+1); if(ack==0) return(0); dat=RevByte(); Ack1(1); stop(); return(dat); } ////////////////////////////////////////////////////////////////// void Delay(unsigned char i) { unsigned char j,k; for(j=i;j>0;j--) for(k=125;k>0;k--); } ///////////////////////////////////////////////////////////////// main() { rw=0; lcden=0; init(); while(1) { switch(AD_CHANNEL) { case 0: PCF8591_SendByte(AddWr,1); D[0]=PCF8591_RcvByte(AddWr); write_shige(0,D[0]); break; case 1: PCF8591_SendByte(AddWr,2); D[1]=PCF8591_RcvByte(AddWr); write_shige(4,D[1]); break; case 2: PCF8591_SendByte(AddWr,3); D[2]=PCF8591_RcvByte(AddWr); write_shige(8,D[2]); break; case 3: PCF8591_SendByte(AddWr,0); D[3]=PCF8591_RcvByte(AddWr); write_shige(12,D[3]); break; case 4: PCF8591_DACONVERSION(AddWr,0,D[4]); break; } D[4]=D[3]; if(++AD_CHANNEL>4) AD_CHANNEL=0; } } ///////////////////////////////////////////////////////////// void write_com(unsigned char com) { rs=0; lcden=0; P0=com; Delay(10); lcden=1; Delay(10); lcden=0; } /////////////////////////////////////////////////////////////// void write_date(unsigned char date) { rs=1; lcden=0; P0=date; Delay(10); lcden=1; Delay(10); lcden=0; } ///////////////////////////////////////////////////////////// void init() { write_com(0x38); write_com(0x0c); write_com(0x06); write_com(0x01); write_com(0x80); } ////////////////////////////////////////////////////////////// void write_shige(unsigned char b,unsigned char m) { unsigned char bai,shi,ge; bai=m/100; shi=m%100/10; ge=m%10; write_com(0x80+0x00+b); write_date(0x30+bai); write_date(0x30+shi); write_date(0x30+ge); } /////////////////////////////////////////////////////////
复制代码