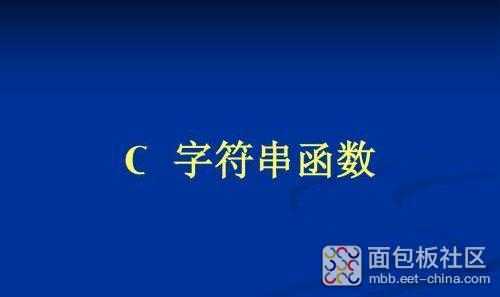
在文章嵌入式Linux开发《C语言专题(五:(3)实用的字符串函数2)》中对C语言中部分字符串函数做了详细介绍,这篇文章将紧跟着前一篇文章详细讲解后续字符串操作函数。
(8)strcasecmp:忽略大小写比较字符串
函数名:strcasecmp
函数原型:int strcasecmp(const char *s1, const char *s2);
功能:忽略大小写比较字符串
返回值:如果忽略大小写比较结束一样,那么这2个字符串相等。如果s1小于s2则比较的结果小于0,如果s1大于s2则比较的结果大于0,如果s1等于s2则比较的结果等于0,所以可以将比较的结果与0比较来判断2个字符串的大小。
说明:比较的方法是对2个字符串中的字符忽略大小写逐个进行比较,直到遇到不匹配(也就是要么大于要么小于)情况就结束。
代码演示:
#include <stdio.h>//一定要加上字符串操作头文件 #include <string.h> //演示字符串忽略大小写比较操作 int main(int argc, char** argv) { char *src = "ab"; char *dest = "AB"; int n = strcasecmp(src, dest); //运行结果:字符串忽略大小写比较ab等于AB if (n == 0) { printf("字符串忽略大小写比较ab等于AB\n"); } else if(n > 0) { printf("字符串忽略大小写比较ab大于AB \n"); } else { printf("字符串忽略大小写比较ab小于AB \n"); } return 0; }
复制代码(9)strncasecmp:忽略大小写比较字符串的前n个字节
函数名:strncasecmp
函数原型: int strncasecmp(const char *s1, const char *s2, size_t n);
功能:忽略大小写比较字符串的前n个字节
返回值:与strcasecmp一样
说明:与strcasecmp一样
代码演示:
#include <stdio.h>//一定要加上字符串操作头文件 #include <string.h> //演示字符串忽略大小写1个字节比较操作 int main(int argc, char** argv) { char *src = "ab"; char *dest = "AB"; int n = strncasecmp(src, dest, 1); //运行结果:字符串忽略大小写比较第一个字节ab等于AB if (n == 0) { printf("字符串忽略大小写比较第一个字节ab等于AB\n"); } else if(n > 0) { printf("字符串忽略大小写比较第一个字节ab大于AB \n"); } else { printf("字符串忽略大小写比较第一个字节ab小于AB \n"); } return 0; }
复制代码(10)strchr:在字符串s中查找指定字符c首次出现的位置
函数名:strchr
函数原型:char *strchr(const char *s, int c);
功能:在字符串s中查找指定字符c首次出现的位置
返回值:如果找到返回在字符串s中第一次出现字符c的位置;如果没有找到,返回NULL
说明:返回的位置是相对于字符串的首地址加上 所查找字符相对于字符串首地址的偏移量
代码演示:
#include <stdio.h>//一定要加上字符串操作头文件 #include <string.h> //演示在字符串中查找指定字符第一次出现的位置 int main(int argc, char** argv) { char *str = "hello world"; //运行结果:str的地址为:0x8048530 //查找字符串str中第一个字母l的位置:0x8048532 //刚好地址相差2个字节 printf("str的地址为:%p \n", str); printf("查找字符串str中第一个字母l的位置:%p \n", strchr(str, 'l')); return 0; }
复制代码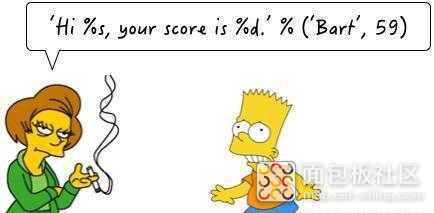
(11)strrchr:在字符串s中反向查找字符c最后一次出现的位置
函数名:strrchr
函数原型:char *strrchr(const char *s, int c);
功能:在字符串s中反向查找字符c最后一次出现的位置
返回值:如果找到返回在字符串s中最后一次出现字符c的位置;如果没有找到返回NULL
说明:如果希望查找某字符在字符串中最后一次出现的位置,可以使用 strrchr() 函数;
代码演示:
#include <stdio.h>//一定要加上字符串操作头文件 #include <string.h> //演示在字符串中查找指定字符最后一次出现的位置 int main(int argc, char** argv) { char *str = "hello world"; //运行结果:str的地址为:0x8048530 //查找字符串str中最后一个字母l的位置:0x8048539 //刚好地址相差9个字节 printf("str的地址为:%p \n", str); printf("查找字符串str中最后一个字母l的位置:%p \n", strrchr(str, 'l')); return 0; }
复制代码(12)strstr:在字符串中查找子字符串第一次出现的位置
函数名:strstr
函数原型:char *strstr(const char *haystack, const char *needle);
功能:在字符串haystack中查找字符串needle第一次出现的位置
返回值:在字符串haystack中查找子字符串needle,如果找到返回haystack中第一次出现needle的位置;如果没有找到,返回NULL。
说明:
代码演示:
#include <stdio.h>//一定要加上字符串操作头文件 #include <string.h> //演示在字符串中查找指定字符串的位置 int main(int argc, char** argv) { char *str = "world"; char *dest = "hello world how are you"; //运行结果:在字符串dest中查找字符串str:world how are you printf("在字符串dest中查找字符串str:%s \n", strstr(dest, str)); return 0; }
复制代码(13)strpbrk:在一个字符串中搜索另一个字符串中的所匹配的任意字符
函数名:strpbrk
函数原型:char *strpbrk(const char *s, const char *accept);
功能:在一个字符串中搜索另一个字符串中的所匹配的任意字符并返回相应的位置
返回值:如果s、accept含有相同的字符,那么返回指向s中第一个相同字符的指针,否则返回NULL。
说明:strpbrk()不会对结束符'\0'进行检索
代码演示:
#include <stdio.h>//一定要加上字符串操作头文件 #include <string.h> //演示在一个字符串中搜索另一个字符串中首次任意字符匹配的位置 int main(int argc, char** argv) { char *str = "world"; char *dest = "hello world how are you"; //运行结果:dest的地址为:0x8048566 //返回字符串中相同字符的位置:0x8048568 内容为: llo world how are you printf("dest的地址为:%p \n", dest); printf("返回字符串中相同字符的位置:%p 内容为: %s \n", strpbrk(dest, str), strpbrk(dest, str)); return 0; }
复制代码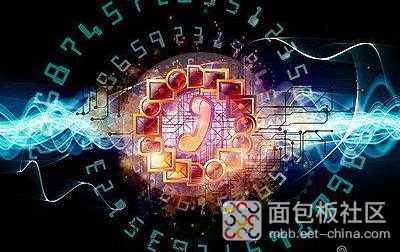
(14)strspn:计算字符串s中连续有几个字符属于字符串 accept
函数名:strspn
函数原型:size_t strspn(const char *s, const char *accept);
功能:计算字符串s中连续有几个字符都属于字符串 accept
返回值:返回字符串str开头连续包含字符串accept内的字符数目。所以,如果str所包含的字符都属于accept,那么返回str的长度;如果str的第一个字符不属于accept,那么返回0
说明:字符是区分大小写;遇到有一个不匹配就结束
代码演示:
#include <stdio.h>//一定要加上字符串操作头文件 #include <string.h> //演示一个字符串中包含另一个字符串中连续内容的个数 int main(int argc, char** argv) { //char *str = "World"; //str中连续有:0 个字符在dest中 //char *str = "world";//str中有连续有:5 个字符在dest中 //注意:这里面是大写字母R 遇到第一个不匹配就结束 char *str = "woRld";//运行结果:str中连续有:2 个字符在dest中 char *dest = "hello world how are you"; printf("str中连续有:%d 个字符在dest中 \n", strspn(str, dest)); return 0; }
复制代码(15)strcspn:计算字符串s中连续有几个字符都不属于字符串 accept
函数名:strcspn
函数原型:size_t strcspn(const char *s, const char *reject);
功能: 与 strspn() 相反,它用来用来计算字符串s中连续有几个字符都不属于字符串accept;
返回值:
说明:字符是区分大小写,遇到有一个匹配就结束
代码演示:
#include <stdio.h>//一定要加上字符串操作头文件 #include <string.h> //演示一个字符串中不包含另一个字符串中连续内容的个数 int main(int argc, char** argv) { //char *str = "world"; //str中连续有:0 个字符不在dest中 //遇到小写字母o就停止 char *str = "WoRLD"; //str中连续有:1 个字符不在dest中 //char *str = "world"; //str中连续有:0 个字符不在dest中 char *dest = "hello world how are you"; //运行结果: printf("str中连续有:%d 个字符不在dest中 \n", strcspn(str, dest)); return 0; }
复制代码(16)atoi:将字符串转换成整型
函数名:atoi
函数原型: int atoi(const char *nptr);
功能:将字符串转换成整型
返回值:返回转换后的整型数;如果不能转换成int或者str为空字符串,那么将返回0
说明:常用在将输入的数字字符串转换成整型 #include <stdlib.h> 不加上这个,直接加上#include <string.h> 也可以
代码演示:
#include <stdio.h>//一定要加上字符串操作头文件 #include <string.h> #include <stdlib.h> //演示字符串转整型 int main(int argc, char** argv) { //char *str = "123.456";//结果为:123 //char *str = "123";//结果为:123 char *str = "1";//结果为:1 printf("结果为:%d \n", atoi(str)); return 0; }
复制代码(17)strtok:根据给定的分割符将字符串分割成一个个小片段字符串
函数名:strtok
函数原型: char *strtok(char *str, const char *delim);
功能:根据给定的分割符将字符串分割成一个个小片段字符串
返回值:返回下一个分割后的字符串指针,如果已经不能再分割则返回NULL
说明:其中参数str不能是字符串常量,否则会出现Segmentation fault (core dumped)
第一次调用时strtok()必须赋予参数str字符串,随后调用将参数str必须设置成NULL;
代码演示:
#include <stdio.h>//一定要加上字符串操作头文件 #include <string.h> //演示根据给定的分界符将字符串分割成一个个小片段字符串 int main(int argc, char** argv) { char str[] = "hello-world:how*are you"; char *dest = "-:*";//按照- : *的方式进行分割 char *p = NULL; printf("%s \n", strtok(str, dest)); /*运行结果: hello world how are you */ while((p = strtok(NULL, dest))) { printf("%s \n", p); } return 0; }
复制代码