我们平时工作休闲之余往往会选择听一些歌曲休闲。但我们是否想过这些音乐播放器是怎么做的呢?你是否想动手试一试呢?
功能
实现音乐播放器的基本功能,可以选择文件播放,也可以拖拉文件播放。实现音乐播放器的常规功能。
本项目中,使用的是.wav文件。
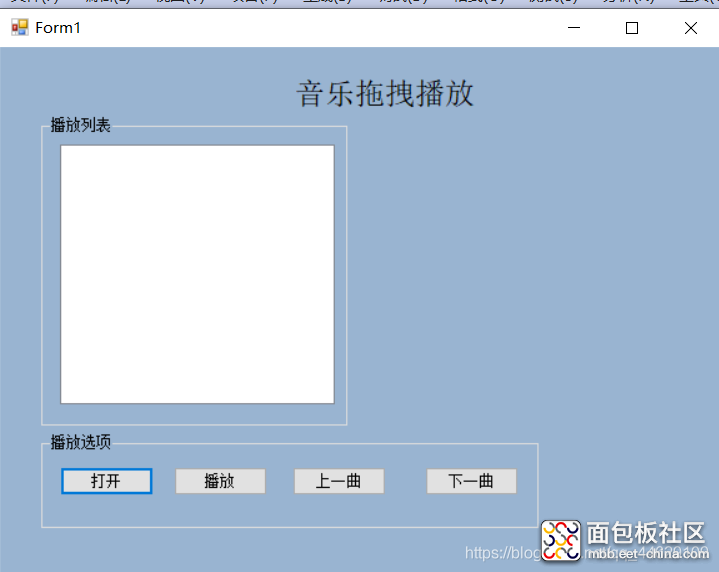
实现
首先。我们需要在VS中建立一个Windows窗体应用工程,自主进行页面设计。
之后进行代码的编写。我将主要的功能代码放在下面,大家可以自主运行。
using System;using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.IO; //Path类用到 using System.Media; //SoundPlayer命名空间 namespace player { public partial class Form1 : Form { public Form1() { InitializeComponent(); } List<string> listsongs = new List<string>(); //用来存储音乐文件的全路径 private void button1_Click(object sender, EventArgs e) { OpenFileDialog ofd = new OpenFileDialog(); ofd.Title = "请选择音乐文件"; //打开对话框的标题 ofd.InitialDirectory = @"F:\music"; //设置打开对话框的初始设置目录 ofd.Multiselect = true; //设置多选 ofd.Filter = @"音乐文件|*.mp3||*.wav|所有文件|*.*"; //设置文件格式筛选 ofd.ShowDialog(); //显示打开对话框 string[] pa_th = ofd.FileNames; //获得在文件夹中选择的所有文件的全路径 for (int i = 0; i < pa_th.Length;i++ ) { listBox1.Items.Add(Path.GetFileName(pa_th[i])); //将音乐文件的文件名加载到listBox中 listsongs.Add(pa_th[i]); //将音乐文件的全路径存储到泛型集合中 } } SoundPlayer sp = new SoundPlayer(); private void listBox1_DoubleClick(object sender, EventArgs e) { SoundPlayer sp = new SoundPlayer(); sp.SoundLocation = listsongs[listBox1.SelectedIndex]; sp.Play(); } private void button2_Click(object sender, EventArgs e) { int index = listBox1.SelectedIndex; //获得当前选中歌曲的索引 index--; if (index <0) { index = listBox1.Items.Count-1; } listBox1.SelectedIndex = index; //将改变后的索引重新赋值给我当前选中项的索引 sp.SoundLocation = listsongs[index]; sp.Play(); } private void button3_Click(object sender, EventArgs e) { int index = listBox1.SelectedIndex; //获得当前选中歌曲的索引 index++; if (index==listBox1.Items.Count) { index = 0; } listBox1.SelectedIndex = index; //将改变后的索引重新赋值给我当前选中项的索引 sp.SoundLocation = listsongs[index]; sp.Play(); } private void Form1_DragEnter(object sender, DragEventArgs e) { if (e.Data.GetDataPresent(DataFormats.FileDrop)) e.Effect = DragDropEffects.Link; else e.Effect = DragDropEffects.None; } private void Form1_DragDrop(object sender, DragEventArgs e) { string[] filePath = (string[])e.Data.GetData(DataFormats.FileDrop); foreach (string file in filePath) { //file就是单个文件路径 SoundPlayer sp = new SoundPlayer(); sp.SoundLocation = file; sp.Play(); // MessageBox.Show(file); } } private void button4_Click(object sender, EventArgs e) { SoundPlayer sp = new SoundPlayer(); if (listBox1.SelectedIndex > 0) sp.SoundLocation = listsongs[listBox1.SelectedIndex]; sp.Play(); } private void groupBox2_Enter(object sender, EventArgs e) { } private void listBox1_SelectedIndexChanged(object sender, EventArgs e) { } private void Form1_Load(object sender, EventArgs e) { } } }
复制代码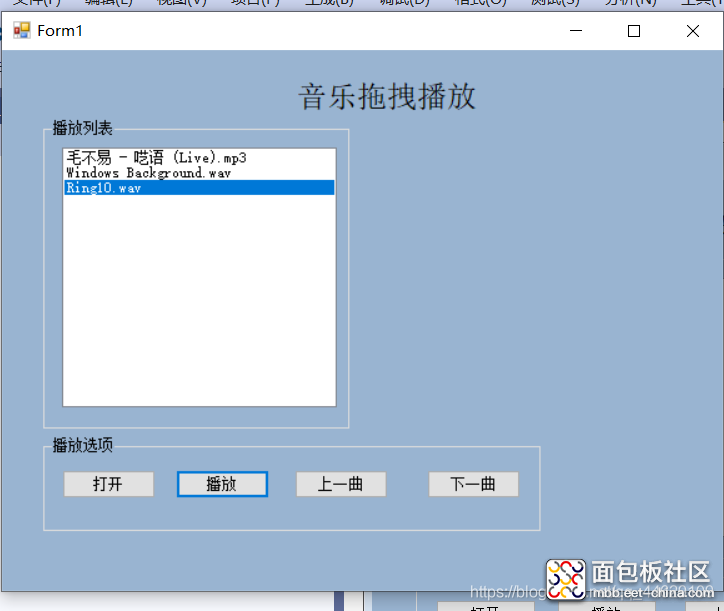